API Based Sign-In
This document describes the API Based Authentication and how it works with MonoSign and External / Central Identity Provider. Suppose we have an application that does not support most of the authentication mechanisms. If this application provides an api for login, then you can use CustomFlow login feature in MonoSign.
curl --location 'www.exampleapp.com/api/login' \
--header 'Key: key-for-api-login' \
--header 'Secret: secret-for-api-login' \
--header 'Content-Type: application/json' \
--data '{
"userName": "userName-For-This-Application"
}'
Example API call for External application
📘 Instructions
Go to Management / Applications / Add New.
Add your application and go to Edit and choose Custom Flow as Login Method under Login tab.
Get the access token / ticket from the target application with this script block, then redirect to the application with the token.
Redirection To MonoSign from External / Central Identity Provider
You can redirect the request started in External / Central Identity Provider to MonoSign. The redirection Url Format is https://{Your-Account-App-Url/}custom-flow/{ApplicationId}?q1=1&q2=2.
Example Url: https://sso.monosign.com/custom-flow/21b3210f-cd30-47d3-97e2-571a1e47ec89?ticket=abc34b789120
All the query string coming from External /Central Identity Provider is passed to CustomFlow part in customFlowModel.Query
variable. This variable holds all query strings as key value in Dictionary<string, string>
.
Custom Flow Coding
# Your application logic for API login
var url = "http://www.example-app.com/api/login";
var clientId = "ClientId-Or-Key";
var clientSecret = "Secret-Or-Master-Password";
var body = new {userName = "userName-To-Login"};
var data = JsonConvert.SerializeObject(body);
var client = new HttpClient()
{
Timeout = TimeSpan.FromSeconds(30)
};
client.DefaultRequestHeaders.Add("client_id", clientId);
client.DefaultRequestHeaders.Add("client_secret", clientSecret);
var content = new StringContent(data, System.Text.Encoding.UTF8, "application/json");
var response = client.PostAsync(url, content).GetAwaiter().GetResult();
var result = response.Content.ReadAsStringAsync().GetAwaiter().GetResult();
var parsed = JObject.Parse(result);
var token = string.Empty;
var message = string.Empty;
if (parsed.ContainsKey("AccessToken"))
{
token = $"{parsed["AccessToken"]}";
}
if (parsed.ContainsKey("Message"))
{
message = $"{parsed["Message"]}";
}
# end of application logic
# MonoSign logic after API call
if (!string.IsNullOrWhiteSpace(token))
{
customFlowResult.RedirectUrl = $"http://www.exampleapp.com/qsToken={token}";
}
else
{
customFlowResult.IsSuccess = false;
customFlowResult.Message = message;
}
Example Custom Flow Code
Steps and Descriptions for API based Sign-In
User Logins to Central Identity Provider and redirects the request to MonoSign with a token / ticket belongs to Central Identity Provider.
MonoSign will take the request and validates the token given by Central Identity Provider.
If token is valid MonoSign makes a API call to External App for “Sign-In” with the extracted userName from the token supplied by Central Identity Provider. If External App successfully sign-ins this request, the token / ticket / sessionId will be taken from the response of External App. This token / ticket / sessionId belongs to the External App. It is not the same token in step 1.
To complete the sign-in process the request is redirected to the External App with given token / ticket / sessionId provided by External App API response.
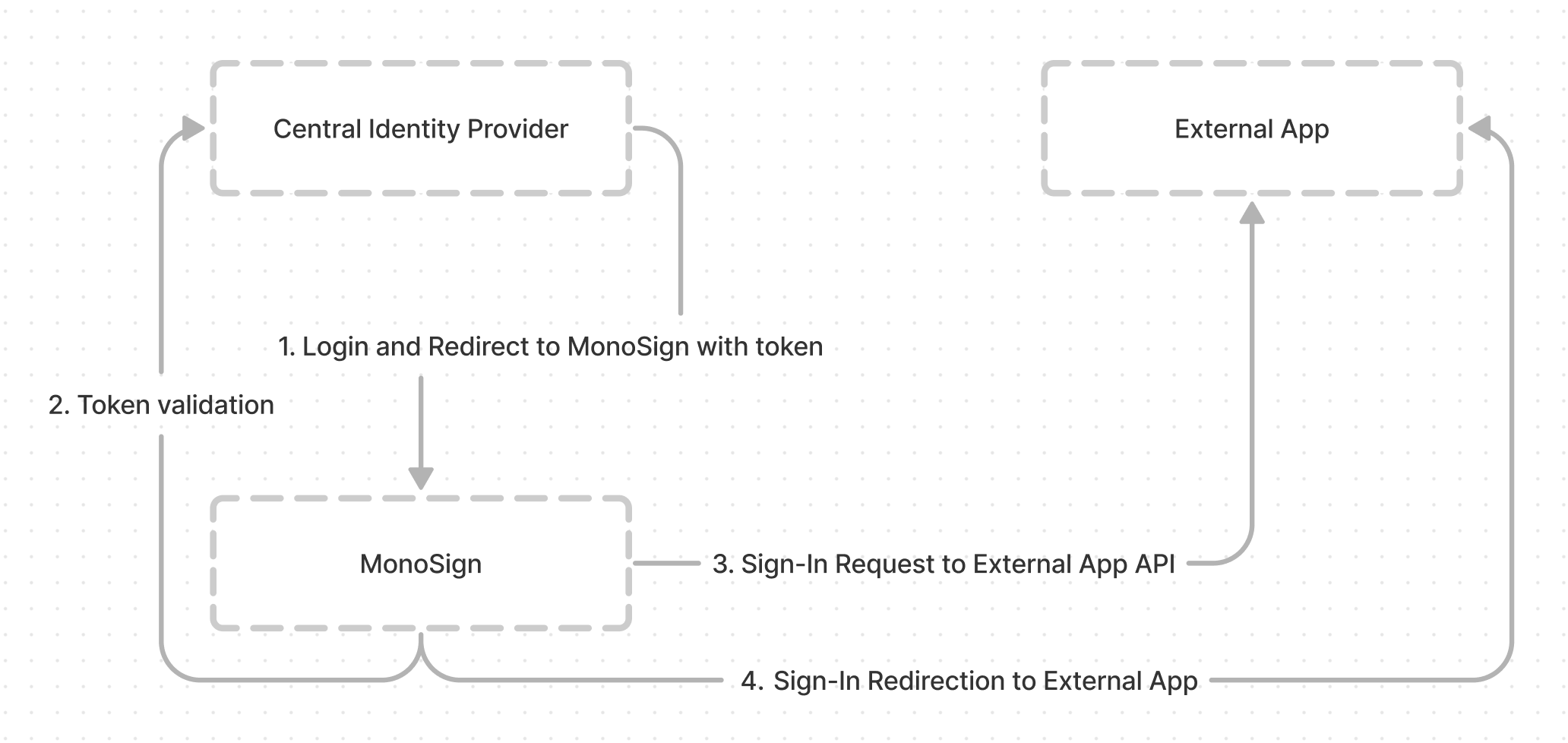
Flow for API Based Sign-In